Python is a powerful and versatile programming language favored by developers for a wide range of applications, from web development to scientific computing. Threading is one of the mechanisms Python provides to achieve concurrency, allowing programs to run multiple operations simultaneously. However, Python’s threading model comes with its limitations, primarily due to the Global Interpreter Lock (GIL). This post will explore the impact of the GIL on threading in Python and discuss strategies to work around its limitations, particularly for CPU-bound tasks.
Understanding the Global Interpreter Lock (GIL)
The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes at once. This means that even in a multi-threaded application, only one thread can execute Python code at a time. The GIL was introduced to simplify the CPython memory management and to make it thread-safe, but it has become a significant bottleneck for CPU-bound and multi-threaded applications.
How the GIL Affects Threading in Python
The primary effect of the GIL on threading is that it restricts multi-threaded programs from achieving true parallelism on multi-core systems. This limitation is particularly problematic for CPU-bound tasks, where the main objective is to maximize CPU usage to improve performance. In such scenarios, the GIL can lead to threads contending for the GIL, resulting in increased overhead and decreased performance.
- I/O-bound vs. CPU-bound: It’s essential to distinguish between I/O-bound and CPU-bound tasks when considering the implications of the GIL. For I/O-bound tasks (waiting for network responses or file I/O), threading can still provide significant performance improvements because the GIL is released while waiting for I/O operations, allowing other threads to run. However, for CPU-bound tasks that require intensive computation, the GIL becomes a bottleneck.
Strategies to Work Around GIL Limitations
Despite the challenges posed by the GIL, there are several strategies developers can employ to minimize its impact and optimize the performance of Python applications.
1. Using Multiprocessing
One of the most effective ways to bypass the limitations of the GIL is to use the multiprocessing
module instead of threading
. Multiprocessing
spawns separate processes for each task, each with its own Python interpreter and, consequently, its own GIL. This approach allows CPU-bound tasks to run in parallel on multiple cores.
from multiprocessing import Pool def cpu_intensive_task(n): # Example CPU-bound task return sum(i*i for i in range(n)) if __name__ == "__main__": with Pool(processes=4) as pool: results = pool.map(cpu_intensive_task, [1000000, 2000000, 3000000, 4000000]) print(results)
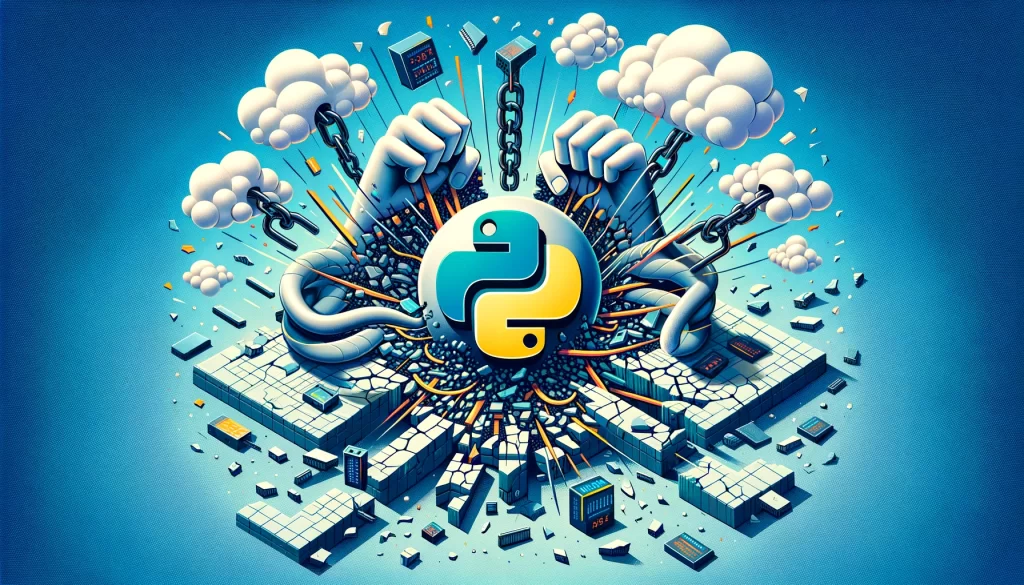
2. Utilizing C Extensions
Another approach to circumventing the GIL is to offload intensive computation to C extensions. Libraries like NumPy use this strategy, executing computationally intensive parts of the code in C, which doesn’t require the GIL. This allows for performance optimizations that are not possible in pure Python.
When discussing the utilization of C extensions as a strategy to circumvent the Global Interpreter Lock (GIL) limitations in Python, particularly for CPU-bound tasks, a classic example is leveraging the NumPy library. NumPy, primarily written in C, is designed for high-performance mathematical and scientific computing. By executing computationally intensive parts of the code at the C level, NumPy operations can run in parallel and without the restrictions imposed by the GIL, leading to significant performance gains.
Example: Utilizing NumPy for Matrix Multiplication
Matrix multiplication is a CPU-intensive operation, especially with large matrices. Using Python’s built-in lists and loops for this task can be inefficient and slow due to the GIL’s limitations. However, NumPy provides a highly optimized implementation of matrix multiplication that takes advantage of C extensions.
First, ensure NumPy is installed in your environment:
pip install numpy
Then, you can perform matrix multiplication using NumPy as follows:
import numpy as np import time # Generate two large matrices matrix_a = np.random.rand(1000, 1000) matrix_b = np.random.rand(1000, 1000) # Record the start time start_time = time.time() # Perform matrix multiplication result = np.dot(matrix_a, matrix_b) # Calculate the elapsed time elapsed_time = time.time() - start_time print(f"Matrix multiplication completed in {elapsed_time:.2f} seconds.")
In this example, np.random.rand(1000, 1000)
generates two 1000×1000 matrices filled with random values. The np.dot
function then performs matrix multiplication between these two matrices. The operation’s speed is significantly enhanced by NumPy’s use of C extensions, bypassing the GIL and utilizing the CPU more efficiently than pure Python code could.
Benefits of Utilizing C Extensions
- Performance: C extensions can execute CPU-bound tasks more efficiently by operating outside the constraints of the GIL.
- Scalability: Operations that leverage C extensions can scale better on multi-core processors, as they are not limited by Python’s single-threaded execution model imposed by the GIL.
- Ecosystem: The Python ecosystem includes numerous libraries that use C extensions for performance-critical tasks, including NumPy for numerical computations, pandas for data manipulation, and more. This allows developers to achieve high performance without needing to write their own C code.
Leveraging C extensions, either by using existing libraries or creating custom ones, can significantly improve the performance of Python applications, especially for CPU-bound tasks. This strategy offers a viable workaround to the limitations imposed by the GIL, enabling developers to harness the full power of their hardware.
3. Employing Concurrent Features
Python 3 introduced the asyncio
library, which provides a single-threaded concurrency model based on coroutines. While asyncio
is mainly beneficial for I/O-bound tasks, it can be used in conjunction with threading
and multiprocessing
to create highly efficient and scalable applications.
Python’s asyncio
library is a cornerstone for writing concurrent code, particularly suited for I/O-bound and high-level structured network code. Using the async/await syntax, asyncio
allows for writing coroutine-based code that is non-blocking and highly efficient, making it an excellent tool for tasks that involve waiting for I/O operations.
Example 1: Asynchronous File Reading with asyncio
In this example, we demonstrate how to perform asynchronous file reading, an I/O-bound task, using asyncio
:
import asyncio async def read_file_async(file_name): async with aiofiles.open(file_name, mode='r') as file: content = await file.read() print(f"File content of {file_name}: {content[:100]}...") # Print first 100 characters async def main(): await asyncio.gather( read_file_async('sample_file_1.txt'), read_file_async('sample_file_2.txt') ) # Running the asyncio event loop asyncio.run(main())
In this example, aiofiles
(an external library that supports asynchronous file operations) is used to open and read files asynchronously. This allows other tasks to run concurrently without being blocked by file I/O operations, demonstrating the efficiency of asyncio
for managing I/O-bound tasks.
Example 2: Concurrent Web Scraping with asyncio
and aiohttp
Here, we use asyncio
in conjunction with aiohttp
for concurrent web scraping, a common I/O-bound scenario:
import asyncio import aiohttp async def fetch_page(session, url): async with session.get(url) as response: print(f"Fetching {url} completed with status {response.status}") return await response.text() async def perform_scraping(urls): async with aiohttp.ClientSession() as session: tasks = [fetch_page(session, url) for url in urls] await asyncio.gather(*tasks) urls = ['http://example.com', 'http://example.org', 'http://example.net'] # Running the event loop asyncio.run(perform_scraping(urls))
This example shows how asyncio
and aiohttp
can be employed to perform HTTP requests concurrently. By using asyncio.gather()
, multiple web pages are fetched simultaneously, greatly improving the efficiency of web scraping operations compared to sequential requests.
Example 3: Asynchronous Database Queries
For database-intensive applications, performing asynchronous queries can significantly enhance responsiveness and throughput:
import asyncio import aiomysql async def query_db(): conn = await aiomysql.connect(host='127.0.0.1', port=3306, user='user', password='password', db='mydb', loop=loop) async with conn.cursor() as cur: await cur.execute("SELECT 42;") print(await cur.fetchone()) conn.close() loop = asyncio.get_event_loop() loop.run_until_complete(query_db())
In this scenario, aiomysql
is utilized to perform non-blocking database queries. This approach ensures that the application remains responsive, even when handling multiple simultaneous database queries or transactions.
These examples demonstrate the versatility and power of employing concurrent features provided by asyncio
for optimizing I/O-bound tasks in Python applications. By leveraging asyncio
, developers can write more efficient, scalable, and responsive applications, overcoming some of the traditional limitations associated with synchronous programming models.
4. Running CPU-bound Tasks in a Separate Process
For applications that are predominantly I/O-bound but have occasional CPU-bound tasks, running those CPU-intensive tasks in a separate process can prevent them from blocking the main application flow. This can be achieved using the concurrent.futures.ProcessPoolExecutor
.
For CPU-bound tasks that are negatively impacted by the Global Interpreter Lock (GIL) in Python, one effective workaround is to run these tasks in separate processes rather than threads. This approach takes advantage of multiple CPU cores, bypassing the GIL and allowing parallel execution of computationally intensive operations. The multiprocessing
module in Python provides a simple API for dividing tasks across multiple processes.
Example: Using multiprocessing
for Parallel Processing
Suppose you have a CPU-intensive task, such as calculating the factorial of a number. By using the multiprocessing
module, you can distribute the task over several processes to utilize multiple cores effectively.
from multiprocessing import Pool def factorial(n): """A CPU-intensive task to calculate the factorial of n.""" if n == 0: return 1 else: return n * factorial(n-1) def calculate_factorials(numbers): """Calculate factorials for a list of numbers in parallel.""" with Pool(processes=4) as pool: results = pool.map(factorial, numbers) print(f"Factorials: {results}") if __name__ == "__main__": numbers = [5, 7, 10, 12] calculate_factorials(numbers)
In this example, the Pool
class is used to create a pool of worker processes. The map
method then applies the factorial
function to each item in the numbers
list, distributing the tasks across the pool. Each process runs in its own Python interpreter with its own GIL, allowing the factorial calculations to proceed in parallel across multiple CPU cores.
Benefits and Considerations
- Performance Gain: This approach can lead to significant performance improvements for CPU-bound tasks, as it allows for true parallel execution on multi-core systems.
- Inter-process Communication: When using multiple processes, it’s important to consider the overhead of inter-process communication. Python objects need to be serialized and deserialized (pickled and unpickled) as they are sent between processes, which can introduce additional overhead.
- Memory Usage: Each process has its own memory space, so running many processes simultaneously can increase the overall memory footprint of your application.
Running CPU-bound tasks in separate processes is a powerful technique for overcoming the limitations of the GIL in Python, making it possible to fully leverage the processing power of multi-core CPUs for improved application performance.
Conclusion
The Global Interpreter Lock poses significant challenges for threading in Python, especially for applications with CPU-bound tasks. However, by understanding the limitations of the GIL and employing strategies like multiprocessing, C extensions, and concurrency features, developers can design applications that maximize performance and efficiency. Experimenting with these approaches in your Python projects can help mitigate the GIL’s impact and unlock new levels of application performance.
We encourage you to share your experiences, questions, or insights on threading and the GIL in the comments below. Whether you’re tackling performance issues in a CPU-bound application or exploring concurrency models, your contributions can enrich our collective understanding and foster further discussion.
No comment