In the vast and intricate world of Python programming, the concept of threading stands as a beacon of efficiency and multitasking. Threading, a form of concurrent execution, allows a program to run multiple operations simultaneously, making optimal use of available resources and significantly improving application performance, especially in I/O-bound and network-driven tasks. With the threading module, Python offers a powerful, high-level interface for threading, enabling developers to create, manage, and deploy threads in their applications seamlessly.
Understanding the threading module is crucial for Python developers aiming to harness the full potential of concurrency in their projects. This module not only simplifies the process of executing multiple operations concurrently but also opens the door to enhancing application responsiveness and overall user experience. Whether you’re building complex web applications, data processing pipelines, or GUI-based software, mastering the threading module allows you to design solutions that are both efficient and scalable. As we delve into the threading module, its key concepts, and practical applications, keep in mind that the knowledge gained here is a stepping stone towards mastering advanced Python programming techniques and unlocking new possibilities in software development.
The threading
Module: Python’s Approach to Thread-Based Parallelism
In the landscape of Python programming, achieving concurrency—running multiple sequences of operations simultaneously—is pivotal for building efficient and responsive applications. One of the primary mechanisms Python offers for such parallel execution is the threading
module, which facilitates thread-based parallelism. This section delves into the threading module, exploring its capabilities, comparing it with other concurrency methods available in Python, and illustrating when threading emerges as the preferable option.
Introduction to the threading
Module
The threading
module provides a high-level interface for threading in Python, allowing for the creation, synchronization, and management of threads. Threads, often considered the smallest unit of processing that can be scheduled by an operating system, enable developers to execute multiple operations concurrently, making applications faster and more interactive.
At its core, the threading
module encapsulates Python’s native thread implementation, offering a variety of classes such as Thread
, Lock
, Condition
, Event
, Semaphore
, and others, to control thread flow and communication. Unlike processes, threads share the same memory space, which makes data sharing among them seamless, though it necessitates careful management to avoid conflicts.
Example Code: Creating and Starting Threads
Here’s a simple example demonstrating how to create and start threads using the threading
module:
import threading def print_numbers(): for i in range(5): print(i) # Creating a thread thread = threading.Thread(target=print_numbers) # Starting the thread thread.start() # Joining the thread ensures it completes execution before the main program continues thread.join()
In this example, the Thread
class is used to create a thread object that runs the print_numbers
function. The start()
method initiates the thread, while join()
is called to ensure that the main program waits for the thread to complete its task.
Comparison with Other Concurrency Methods in Python
Python offers several mechanisms for achieving concurrency, including threading, multiprocessing, asyncio, and concurrent.futures. Each has its strengths and suitable use cases:
- Multiprocessing: Best suited for CPU-bound tasks that require parallel execution across multiple CPU cores. Unlike threading, multiprocessing bypasses the Global Interpreter Lock (GIL) by creating separate memory spaces for each process.
- Asyncio: Provides an event loop to manage asynchronous I/O operations, ideal for high I/O-bound tasks without the traditional overhead of threads or processes.
- Concurrent.futures: A high-level interface for asynchronously executing callables, abstracting over both threads (
ThreadPoolExecutor
) and processes (ProcessPoolExecutor
).
Threading is particularly advantageous in scenarios where I/O-bound tasks predominate, such as network communication, file I/O, or user interface responsiveness. In these cases, the overhead of managing multiple threads is offset by the efficiency gains from concurrent I/O operations. Additionally, threading is beneficial when maintaining shared state or context among concurrently executing tasks is necessary, leveraging the shared memory space of threads.
When to Use Threading
Threading should be considered when developing applications that require concurrent execution of I/O-bound tasks, need to maintain responsiveness in user interfaces, or when the overhead of process creation and inter-process communication outweighs the benefits of parallel CPU computation. Despite the constraints posed by the GIL in CPU-bound operations, the threading
module remains a powerful tool for specific concurrency needs in Python, providing a balance between simplicity and functionality for managing parallel execution.
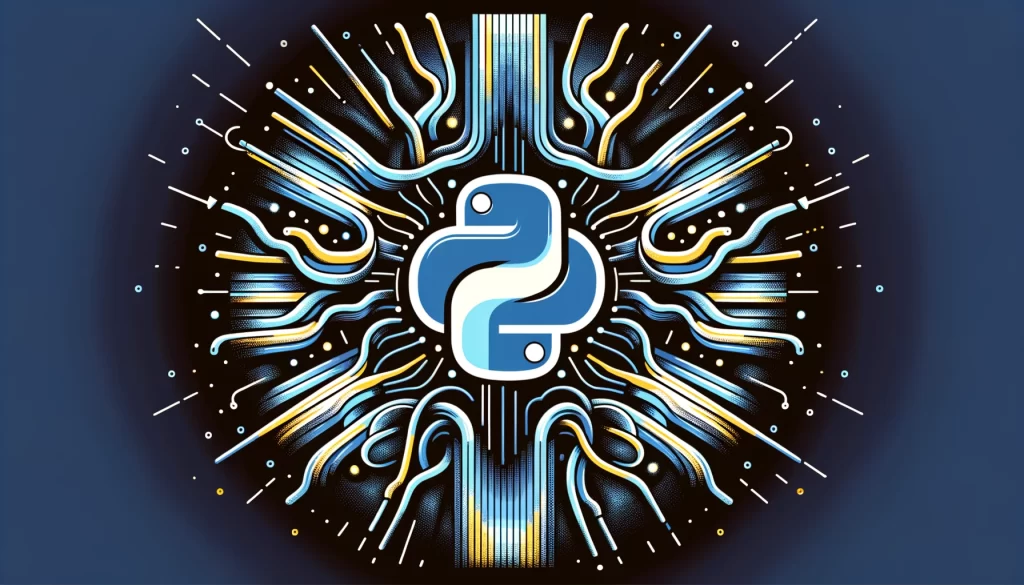
Key Concepts in Threading
Threading in Python is facilitated by the threading
module, which provides a way to perform multiple operations concurrently, making applications more efficient and responsive. Understanding the foundational concepts of threading is essential for leveraging its full potential. This section explores the definition of threads, the nature of thread objects, and the life cycle of a thread in Python.
Definition and Explanation of Threads in Python
A thread, in the context of Python, is the smallest unit of execution that can be scheduled by the operating system. It is a sequence of instructions that can run independently of other threads, allowing for concurrent execution of code. Python’s threading model allows developers to create programs that can handle multiple tasks at once, improving the application’s overall throughput and responsiveness.
Threads share the same memory space within a process, enabling them to access the same data and resources. However, this shared access necessitates careful synchronization to prevent data corruption or conflicts.
Thread Objects: The Basic Building Blocks of Threading
The threading
module in Python introduces the concept of thread objects, which encapsulate the behavior and state of individual threads. A thread object is an instance of the Thread
class provided by the threading
module. It represents an activity that is run in a separate thread of control. There are two main ways to specify the activity: by passing a callable object to the constructor, or by overriding the run()
method in a subclass.
Example: Creating a Thread Object
import threading def worker(): """Thread worker function""" print('Worker') # Create a thread object that will run the worker function thread = threading.Thread(target=worker)
Life Cycle of a Thread: Creating, Starting, Executing, and Terminating Threads
The life cycle of a thread in Python encompasses several stages:
- Creating: A thread is created by instantiating the
Thread
class with a target function that it will execute. - Starting: The thread’s execution begins when its
start()
method is called. This method invokes the target function in a separate thread of control. - Executing: After starting, the thread is executed, performing the operations defined in its target function or
run()
method. During this phase, the thread can interact with other threads and access shared resources, necessitating synchronization mechanisms like locks to prevent conflicts. - Terminating: A thread terminates when its target function completes. A thread can also be terminated prematurely by raising an exception.
Threads can also be explicitly joined using the join()
method, which blocks the calling thread until the thread whose join()
method is called is terminated. This is useful for waiting for a thread to complete its tasks before proceeding with other operations that depend on the thread’s output.
Example: Starting and Joining a Thread
# Start the thread thread.start() # Wait for the thread to complete thread.join() print('Thread has finished execution.')
Understanding these key concepts in threading is vital for developing multi-threaded applications in Python. It enables developers to write code that efficiently manages concurrent operations, leading to more performant and scalable software solutions.
Managing Threads in Python
Effectively managing threads is crucial for leveraging the full potential of Python’s threading capabilities. This involves not just initiating and coordinating thread execution but also understanding the nuances of different thread types. In this section, we’ll cover how to start threads, the importance of joining threads to synchronize their execution, and the distinction between daemon and non-daemon threads.
Starting Threads
To start a thread in Python, you first need to create a Thread
instance from the threading
module, specifying the target function that the thread will execute. Starting a thread is as simple as calling its start()
method, which schedules the thread for execution by the operating system.
Example: Initiating a Thread
import threading def print_numbers(): for i in range(5): print(i) # Creating a thread object that targets the print_numbers function number_thread = threading.Thread(target=print_numbers) # Initiating the thread number_thread.start()
Once start()
is called, the thread becomes alive and begins executing its target function.
Joining Threads
The join()
method of a thread is a mechanism to allow one thread to wait for another to finish its execution. This is particularly useful when the flow of your program depends on the completion of various threads. By calling join()
, you ensure that the main thread (or any other thread) waits for the specified thread to complete before proceeding.
Example: Joining a Thread
# Continuing from the previous example # Waiting for the thread to complete number_thread.join() print("Thread execution complete.")
Using join()
guarantees that the main program only continues once the thread has finished its task, maintaining the desired sequence of operations.
Daemon vs. Non-Daemon Threads
In Python threading, threads can be classified into two types: daemon and non-daemon. The main difference lies in their lifecycle and how they are terminated.
- Non-Daemon Threads: By default, threads are non-daemon, meaning the program will wait for these threads to complete before it terminates. These threads are suitable for tasks that must be completed before the program ends.
- Daemon Threads: Daemon threads are set to run in the background and are killed as soon as all non-daemon threads have completed. They are useful for tasks that run continuously in the background, such as monitoring or server processes that should not prevent the program from exiting.
Setting a Thread as Daemon
background_thread = threading.Thread(target=background_task) background_thread.daemon = True background_thread.start()
Choosing between daemon and non-daemon threads depends on your application’s requirements and the nature of the task being performed by the thread. It’s important to carefully consider the use of daemon threads, as they can be abruptly terminated, potentially leaving resources in an inconsistent state.
By mastering the initiation, synchronization, and classification of threads, developers can effectively manage concurrent operations in Python, leading to more efficient and responsive applications.
Synchronization and Locks
In multithreaded applications, ensuring that threads interact with shared data or resources in a safe and predictable manner is crucial. Without proper management, concurrent access to shared data can lead to race conditions, where the output depends on the non-deterministic ordering of threads’ execution. This section delves into thread synchronization in Python and introduces various mechanisms, including Locks, RLocks, Semaphores, and Conditions, designed to facilitate safe thread execution.
Introduction to Thread Synchronization
Thread synchronization is a technique used to control the execution order of threads to prevent race conditions. It ensures that only one thread can access a critical section of code or data at any given time, thus preserving the integrity of shared resources. Synchronization is typically achieved through the use of locking mechanisms that block access to the resources for all but one thread.
Using Locks
A Lock is the most fundamental synchronization mechanism provided by Python’s threading
module. It allows a thread to lock a resource, perform operations, and then release the lock. While the lock is held, any other thread attempting to acquire the lock will be blocked until the lock is released.
Example: Locking Mechanism
import threading # Creating a lock object lock = threading.Lock() # Acquiring the lock lock.acquire() # Critical section of code that modifies shared data # ... # Releasing the lock lock.release()
It’s recommended to use the lock in a with
statement to ensure it’s always released, even if an exception occurs:
with lock: # Critical section of code pass
Using RLocks
RLock (Reentrant Lock) is similar to Lock, with the main difference being that it allows a thread to acquire the lock multiple times without blocking. This is useful in scenarios where a thread needs to enter a critical section of code that’s already protected by the same lock.
Using Semaphores
A Semaphore is a more advanced locking mechanism that allows a specified number of threads to access a critical section. It’s useful when you need to limit access to a resource that can support a limited number of simultaneous accesses.
Example: Semaphore
# Creating a semaphore that allows up to two threads to access the critical section semaphore = threading.Semaphore(2) with semaphore: # Critical section that can be accessed by up to two threads simultaneously pass
Using Conditions
A Condition is a synchronization primitive that allows threads to wait for certain conditions to be met. Conditions are based on Locks and can be used to pause a thread’s execution until notified by another thread.
Example: Condition
import threading # Creating a condition object condition = threading.Condition() with condition: # Wait for the condition to be met condition.wait() # Critical section that executes once the condition is met pass # Another thread with condition: # Notify other threads that the condition has been met condition.notifyAll()
Thread synchronization and the correct use of locks, semaphores, and conditions are fundamental to writing safe and reliable multithreaded Python applications. By preventing race conditions and ensuring controlled access to shared resources, these mechanisms help maintain data integrity and application stability.
Conclusion
Through our exploration of threading in Python, we’ve delved into the foundational aspects of managing and synchronizing threads to ensure efficient and safe concurrent execution. Starting from the creation and management of threads with the threading
module, to understanding the vital role of synchronization mechanisms like Locks, RLocks, Semaphores, and Conditions, we’ve covered the essential ground to empower Python developers to leverage threading in their applications effectively.
The ability to manage threads properly and synchronize access to shared resources is crucial for developing robust multithreaded applications. These mechanisms help prevent race conditions and ensure that data integrity is maintained across concurrent executions. As we’ve seen, Python offers a variety of tools within the threading
module to address these challenges, providing a flexible and powerful way to implement concurrency.
As we move forward, our journey into threading in Python will take us into the realm of practical applications and considerations. In the upcoming sections, we’ll focus on:
- Use Cases for Threading: We’ll explore how threading can significantly improve the performance of I/O-bound tasks and enhance UI responsiveness, showcasing real-world scenarios where multithreading shines.
- Limitations of Threading Due to the GIL: A deep dive into the Global Interpreter Lock (GIL) and its impact on threading in Python will reveal the challenges it poses, especially for CPU-bound tasks, and how to navigate them.
- Advanced Threading Techniques: Building on the basics, we’ll examine more sophisticated threading strategies and how to employ them to solve complex problems in Python applications.
By mastering these advanced concepts and techniques, developers can unlock the full potential of threading in Python, crafting applications that are not only efficient and responsive but also maintainable and scalable. Stay tuned as we continue to unravel the intricacies of threading, equipping you with the knowledge to tackle even the most demanding concurrent programming challenges.
No comment