Python, a versatile and widely-used programming language, boasts a rich set of data types that are foundational to its ease of use and flexibility. At the heart of Python’s popularity is its readability and simplicity, making it an ideal choice for beginners and professionals alike. Understanding Python’s data types is not just a stepping stone but a crucial element for effective programming. From simple types like integers and strings to more complex structures like lists and dictionaries, each data type in Python is designed to handle specific kinds of data efficiently. This guide aims to delve deep into each of these types, offering a comprehensive understanding that is essential for anyone looking to master Python.
Our exploration begins with the basic data types: integers, floats, and strings. These are the building blocks of Python programming. Integers and floats handle numerical data, whereas strings are used for text. We will discuss how these types are defined and manipulated in Python, including operations like addition, subtraction, and concatenation. You’ll learn about the subtleties of each type, such as how Python handles large integers or the intricacies of string formatting. Additionally, this section will cover Boolean values and Python’s dynamic typing, which allows for a more flexible approach to coding but also demands a clear understanding to avoid common pitfalls.
Moving beyond the basics, we will explore complex data types such as lists, tuples, sets, and dictionaries. These types are incredibly powerful, enabling programmers to organize and process large volumes of data effectively. Lists and tuples are perfect for ordered collections of items, while sets are ideal for unique elements and fast membership testing. Dictionaries, with their key-value pairs, are indispensable for representing real-world data in a structured manner. For each of these types, we will provide practical usage examples, highlight their distinct characteristics, and discuss the typical scenarios where they are most useful. Common mistakes and best practices will also be addressed, ensuring that you not only understand what these data types are but also how to use them correctly and efficiently in your Python programs.
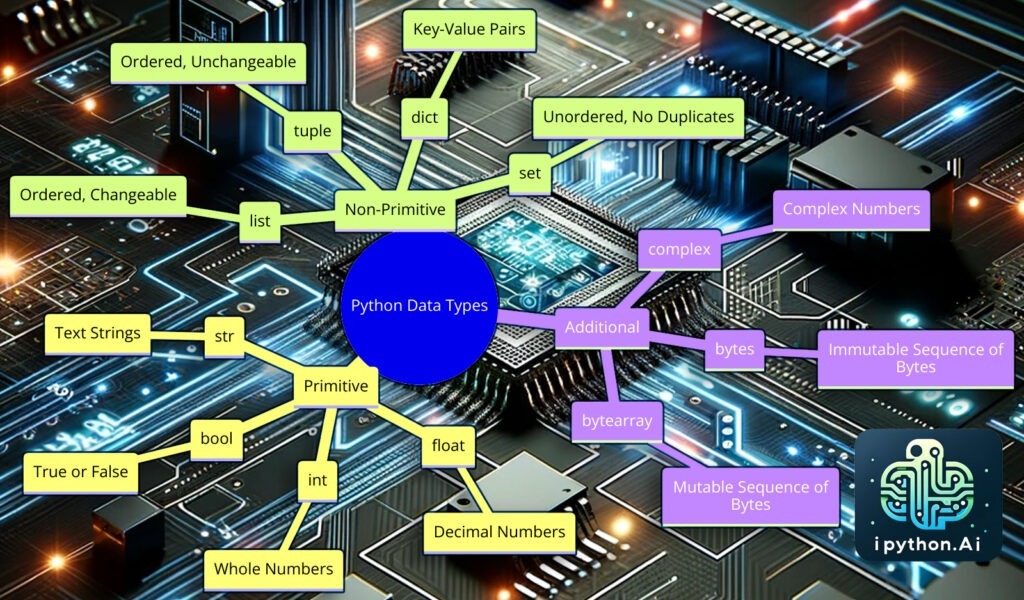
Through this comprehensive guide, we aim to equip you with a solid understanding of Python’s data types, paving the way for more advanced programming concepts and effective problem-solving skills in Python.
Integer
Explanation: Integers are whole numbers without a fractional part.
Usage:
- Counting items
- Loop iterations
- Simple arithmetic operations
Example:
count = 5 for i in range(count): print(i)
Tips:
- Use integers for countable items.
- Be cautious with integer division, as it truncates decimal parts.
Common Mistakes:
- Overlooking integer overflow in other languages (Python handles large integers gracefully).
Float
Explanation: Floats represent real numbers and include decimal points.
Usage:
- Representing currency values
- Scientific calculations
Example:
price = 19.99 discount = price * 0.2 print(discount)
Tips:
- For precise decimal arithmetic, consider using the
decimal
module.
Common Mistakes:
- Assuming float arithmetic is always precise (floating-point errors can occur).
String
Explanation: Strings are sequences of characters.
Usage:
- Text processing
- Storing names, messages
Example:
greeting = "Hello, Python!" print(greeting[7:])
Tips:
- Use triple quotes for multi-line strings.
- Remember strings are immutable.
Common Mistakes:
- Forgetting to escape special characters like backslashes or quotes.
List
Explanation: Lists are ordered, mutable collections.
Usage:
- Storing a sequence of items
- Iterating through elements
Example:
fruits = ["apple", "banana", "cherry"] fruits.append("date") print(fruits)
Tips:
- Use list comprehensions for concise and readable transformations.
- Lists are great for stack or queue implementations.
Common Mistakes:
- Modifying a list while iterating over it.
Tuple
Explanation: Tuples are immutable sequences.
Usage:
- Storing data that shouldn’t change
- Tuple unpacking
Example:
coordinates = (10, 20) x, y = coordinates
Tips:
- Use tuples for fixed data sets.
- Tuples are hashable and can be used as keys in dictionaries.
Common Mistakes:
- Trying to modify a tuple.
Dictionary
Explanation: Dictionaries hold key-value pairs.
Usage:
- Storing and retrieving data by keys
- Implementing mappings
Example:
person = {"name": "Alice", "age": 25} print(person["name"])
Tips:
- Use
dict.get(key)
to avoidKeyError
exceptions. - Dictionary comprehensions can create dictionaries dynamically.
Common Mistakes:
- Using mutable types as dictionary keys.
Boolean
Explanation: Booleans represent True
or False
.
Usage:
- Conditional statements
- Representing state
Example:
is_active = True if is_active: print("Active") else: print("Inactive")
Tips:
- Use Boolean expressions directly in conditions (avoid unnecessary
if
checks). - Understand truthy and falsy values in Python.
Common Mistakes:
- Misunderstanding Python’s evaluation of truthy and falsy values.
Conclusion
Understanding Python’s data types is fundamental to writing effective and efficient code. By following the tips and best practices outlined, you’ll avoid common pitfalls and enhance your coding skills.
No comment