Variables are the cornerstone of programming, and Python, known for its simplicity and readability, utilizes them effectively. This comprehensive guide delves into Python variables, exploring their functionality, key concepts, and rules.
1. Scope of Variables
Scope determines where a variable can be accessed within a program. Python has two primary scopes:
- Local Scope: Variables declared within a function are local to that function. They can’t be accessed outside of it. For example:
def my_function(): local_var = 5 # Local variable print(local_var) # Accessible here # print(local_var) # Error if uncommented, not accessible here
- Global Scope: Variables declared outside any function have a global scope, accessible anywhere in the code.
global_var = 10 # Global variable def my_function(): print(global_var) # Accessible here print(global_var) # Also accessible here
Python lacks block scope, a feature in languages like JavaScript.
2. Constants
Constants are variables meant to remain unchanged. Python doesn’t have a built-in constant type, but the convention is to use uppercase letters:
MAX_SPEED = 120 PI = 3.14159
3. Naming Rules
Variables in Python must adhere to certain rules:
- Cannot start with a number.
- No special symbols, except underscore (_).
- Cannot be Python reserved keywords (like
if
,for
,class
).
4. Naming Conventions
Common practices include:
snake_case
for variables and functions.CamelCase
for class names._variable
(leading underscore) for indicating non-public variables.
5. Data Types
Python supports various data types:
int
: Integer, e.g.,age = 21
float
: Floating-point number, e.g.,price = 19.99
str
: String, e.g.,name = "Alice"
bool
: Boolean (True or False), e.g.,is_valid = True
- Collection types:
list
,tuple
,dict
6. Variable Declaration and Initialization
Variables are created by assignment:
x = 5 # Creates an integer variable 'x'
7. Variable Lifetime
Depends on scope:
- Local variables: Exist during function execution.
- Global variables: Last throughout program runtime.
8. Environment Variables
Used for configuration, accessed via os.environ
:
import os db_password = os.environ.get('DB_PASSWORD')
9. Mutable and Immutable Variables
- Immutable types (int, float, tuple) can’t be altered once created.
- Mutable types (lists, dictionaries) can be modified.
10. Variable Hoisting
Not applicable in Python. Variables are available only after definition.
11. Static and Dynamic Typing
Python is dynamically typed, the type is inferred at runtime:
var = "Hello" # Initially a string var = 15 # Now an integer
12. Reference and Value Types
Variables reference objects in memory. This is crucial for understanding interactions in mutable and immutable types.
Additional Concepts
- Type Hinting: Optional type hints introduced in Python 3.5 for readability and debugging
def greet(name: str) -> str: return "Hello " + name
- Unpacking: Directly unpack collections into variables:
squares = [x**2 for x in range(10)]
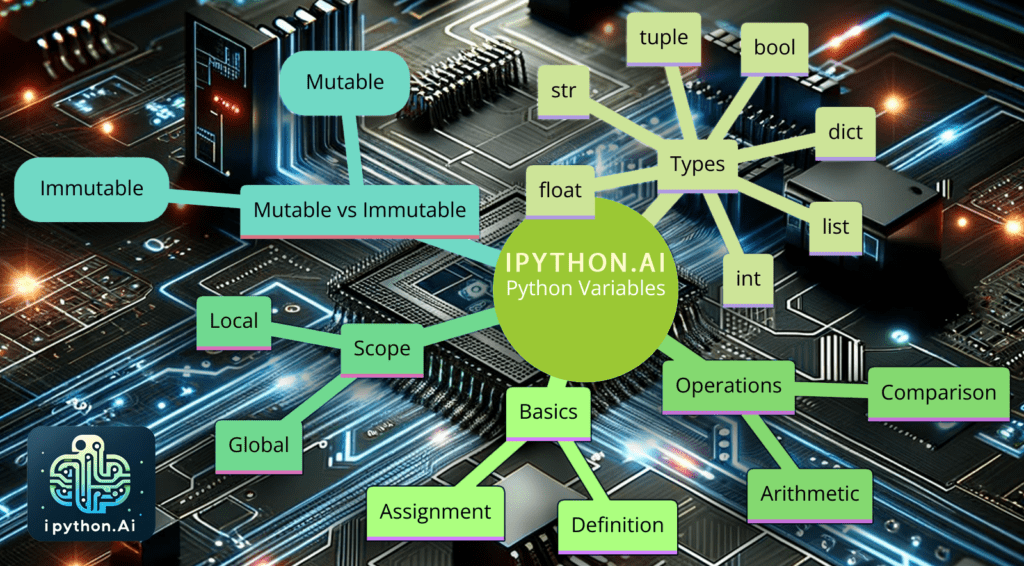
Conclusion
Mastering variables in Python is essential for effective programming. Understanding their scope, types, and best practices enables you to leverage Python’s capabilities fully, whether you’re a beginner or an experienced coder.
No comment